ローディングをまとめてみました。
おしゃれなサイトさんはローディングを組み込んでいるなー、と思う今日この頃です。
意外と簡単なのでお時間ありましたら組み込んでみてくださいませ。
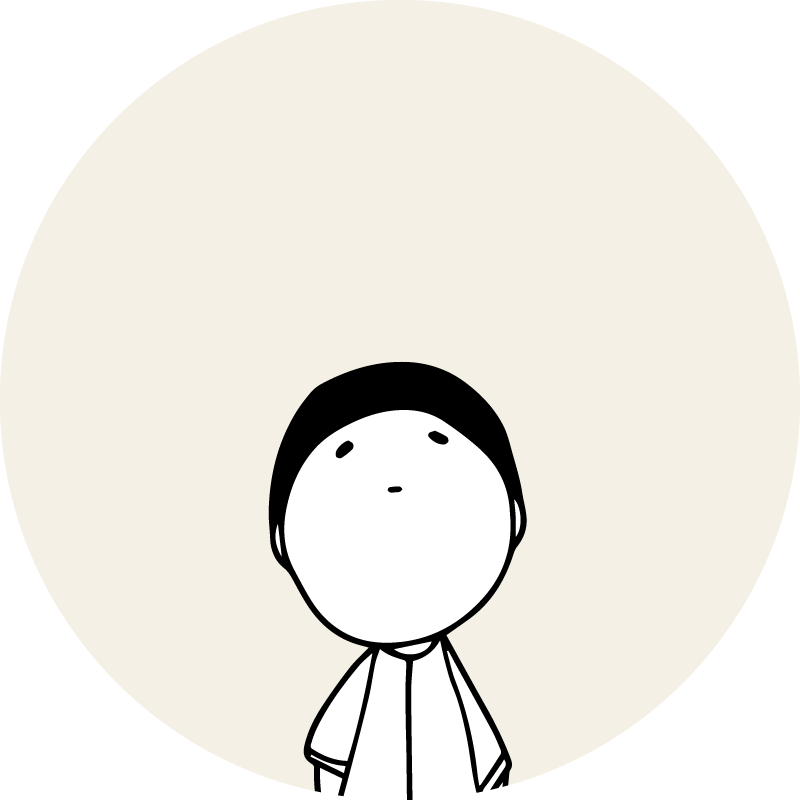
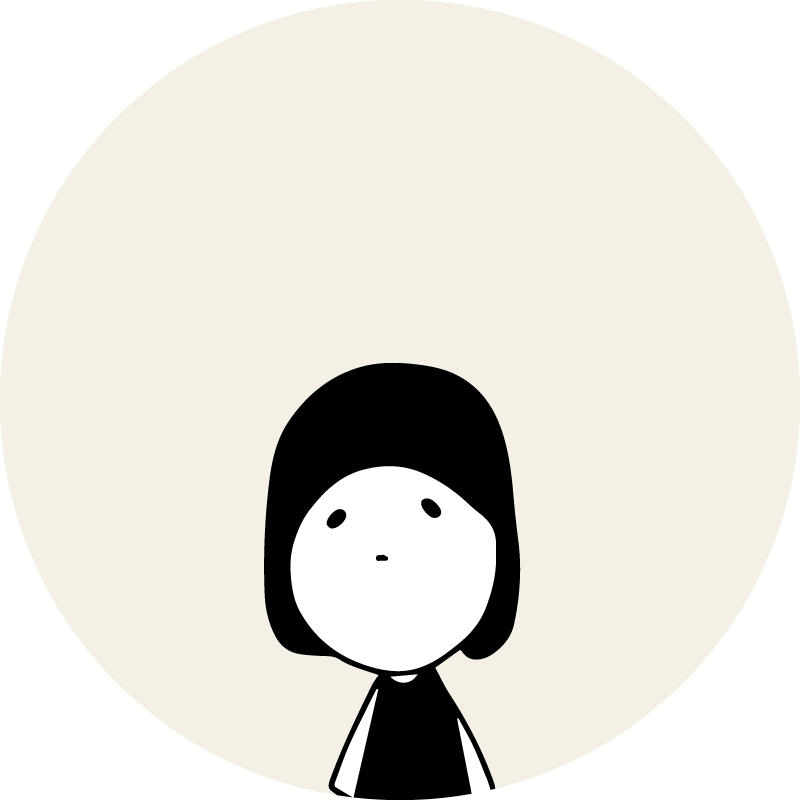
【サンプル】ローディング(テキストだけでふわっと)
シンプルにテキストとちょっとした動きだけのローディングです。
See the Pen 【jQuery】 ローディング(テキストだけでふわっと) by 125naroom (@125naroom) on CodePen.
Rerunでローディングを確認できます▲
HTMLとCSSとjQueryはこちら
HTML
<div id="loading">
<div id="loading_box">
<div class="loading-one animation_loading">
<p class="loading-txt">Loading</p>
</div>
</div>
</div>
CSS
#loading {
position: fixed;
top: 0;
left: 0;
bottom: 0;
z-index: 99999;
width: 100%;
height: 100%;
background: #999;
}
#loading_box {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
width: 100%;
text-align: center;
}
.loading-one {
display: inline-block;
border-top: 1px solid #ffffff;
border-bottom: 1px solid #ffffff;
}
.loading-one p.loading-txt {
color: #fff;
font-size: 20px;
letter-spacing: 0.25em;
line-height: 2.0;
padding: 2em 0;
}
jQuery
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
//ローディング画面の表示
$(window).on('load',function(){
$("#loading").delay(1500).fadeOut('slow');//ローディング画面を1.5秒(1500ms)待機してからフェードアウト
$("#loading_box").delay(1200).fadeOut('slow');//ローディングテキストを1.2秒(1200ms)待機してからフェードアウト
});
【サンプル】ローディング(数字カウントアップ)
※ 数字カウントアップは、progressbarのJSを追加する必要があります。
See the Pen 【jQuery】 ローディング(数字カウントアップ) by 125naroom (@125naroom) on CodePen.
Rerunでローディングを確認できます▲
HTMLとCSSとjQueryはこちら
HTML
<div id="loading">
<div id="loading_text"></div>
</div>
CSS
#loading {
position: fixed;
top: 0;
left: 0;
bottom: 0;
width: 100%;
height: 100%;
z-index: 99999;
background: #222222;
text-align: center;
}
jQuery
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/progressbar.js/1.1.0/progressbar.min.js"></script>
//テキストのカウントアップの設定
var bar = new ProgressBar.Line(loading_text, {//id名を指定
strokeWidth: 0,//進捗ゲージの太さ
duration: 1000,//時間指定(1000=1秒)
trailWidth: 0,//線の太さ
text: {//テキストの形状を直接指定
style: {
position:'absolute',
left:'50%',
top:'50%',
margin:'0',
transform:'translate(-50%,-50%)',
'font-family':'sans-serif',
'font-size':'1.5rem',
color:'#fff',
},
autoStyleContainer: false //自動付与のスタイルを切る
},
step: function(state, bar) {
bar.setText(Math.round(bar.value() * 100) + ' %'); //テキストの数値
}
});
//アニメーションスタート
bar.animate(1.0, function () {//バーを描画する割合を指定します 1.0 なら100%まで描画
$("#loading").delay(500).fadeOut(800);//アニメーションが終わったら#loadingをフェードアウト
});
【サンプル】ローディング(画像をふわっと)
See the Pen 【jQuery】 ローディング(画像をふわっと) by 125naroom (@125naroom) on CodePen.
Rerunでローディングを確認できます▲
HTMLとCSSとjQueryはこちら
HTML
<div id="loading">
<div class="kvArea" id="loading_box">
<div class="img_box"><img src="https://125naroom.com/demo/img/kinoko_001.png" alt="" class="fadeUp"></div>
</div>
</div>
CSS
#loading {
position: fixed;
top: 0;
left: 0;
bottom: 0;
width: 100%;
height: 100%;
z-index: 99999;
background: #fff;
text-align: center;
}
#loading_box {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
#loading .kvArea {
width: 100%;
}
#loading .kvArea .img_box {
text-align: center;
}
#loading .kvArea .img_box img {
max-width: 100%;
height: auto;
}
.fadeUp {
animation-name: fadeUpAnime;
animation-duration: 1.2s;
animation-delay: 1.2s;
animation-fill-mode: forwards;
opacity: 1;
}
@keyframes fadeUpAnime {
from {
opacity: 1;
transform: translateY(0);
}
to {
opacity: 0;
transform: translateY(-100px);
}
}
jQuery
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
//ローディング画面の表示
$(window).on('load',function(){
$("#loading").delay(1500).fadeOut('slow');//ローディング画面を1.5秒(1500ms)待機してからフェードアウト
$("#loading_box").delay(1200).fadeOut('slow');//ローディング画像を1.2秒(1200ms)待機してからフェードアウト
});
【サンプル】ローディング(画像と数字カウントアップ)
※ 数字カウントアップは、progressbarのJSを追加する必要があります。
See the Pen 【jQuery】 ローディング(画像と数字カウントアップ) by 125naroom (@125naroom) on CodePen.
Rerunでローディングを確認できます▲
HTMLとCSSとjQueryはこちら
HTML
<div id="loading">
<div class="kvArea" id="loading_logo">
<div class="img_box"><img src="https://125naroom.com/demo/img/kinoko_001.png" alt="" class="fadeUp"></div>
</div>
<div id="loading_text"></div>
</div>
CSS
#loading {
position: fixed;
top: 0;
left: 0;
bottom: 0;
width: 100%;
height: 100%;
z-index: 99999;
background: #fff;
text-align: center;
}
#loading_logo {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
#loading .kvArea {
width: 100%;
}
#loading .kvArea .img_box {
text-align: center;
}
#loading .kvArea .img_box img {
max-width: 100%;
height: auto;
}
.fadeUp {
animation-name: fadeUpAnime;
animation-duration: 1.2s;
animation-delay: 1.2s;
animation-fill-mode: forwards;
opacity: 1;
}
@keyframes fadeUpAnime {
from {
opacity: 1;
transform: translateY(0);
}
to {
opacity: 0;
transform: translateY(-100px);
}
}
jQuery
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script type="text/javascript" src="https://cdnjs.cloudflare.com/ajax/libs/progressbar.js/1.1.0/progressbar.min.js"></script>
//ローディング画面の表示
$(window).on('load',function(){
$("#loading").delay(1200).fadeOut('slow');//ローディング画面を1.2秒(1200ms)待機してからフェードアウト
});
//テキストのカウントアップの設定
var bar = new ProgressBar.Line(loading_text, {//id名を指定
strokeWidth: 0,//進捗ゲージの太さ
duration: 1000,//時間指定(1000=1秒)
trailWidth: 0,//線の太さ
text: {//テキストの形状を直接指定
style: {
position:'absolute',
left:'50%',
top:'80%',
margin:'0',
transform:'translate(-50%,-50%)',
'font-family':'sans-serif',
'font-size':'1.5rem',
color:'#333',
},
autoStyleContainer: false //自動付与のスタイルを切る
},
step: function(state, bar) {
bar.setText(Math.round(bar.value() * 100) + ' %'); //テキストの数値
}
});
//アニメーションスタート
bar.animate(1.0, function () {//バーを描画する割合を指定します 1.0 なら100%まで描画
$("#loading_text").delay(0).fadeOut('fast');//アニメーションが終わったら#loading_textをフェードアウト
});
メモ
jQueryのことで何かわからないことがあればjQueryの日本語リファレンスサイトがあるので一度チェックしてみるといろいろ解決できたりしますよ。
さいごに
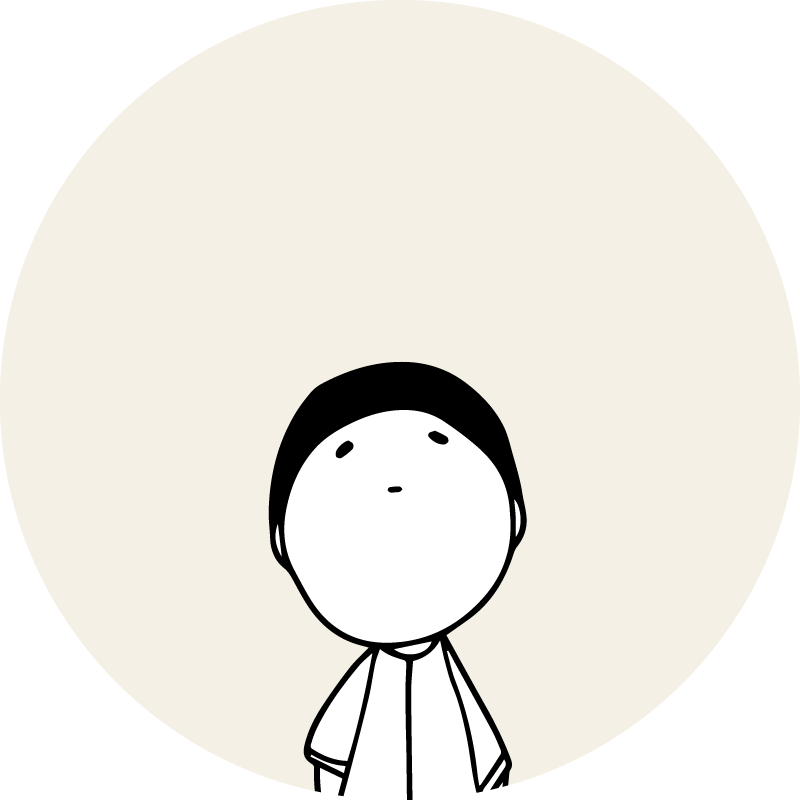
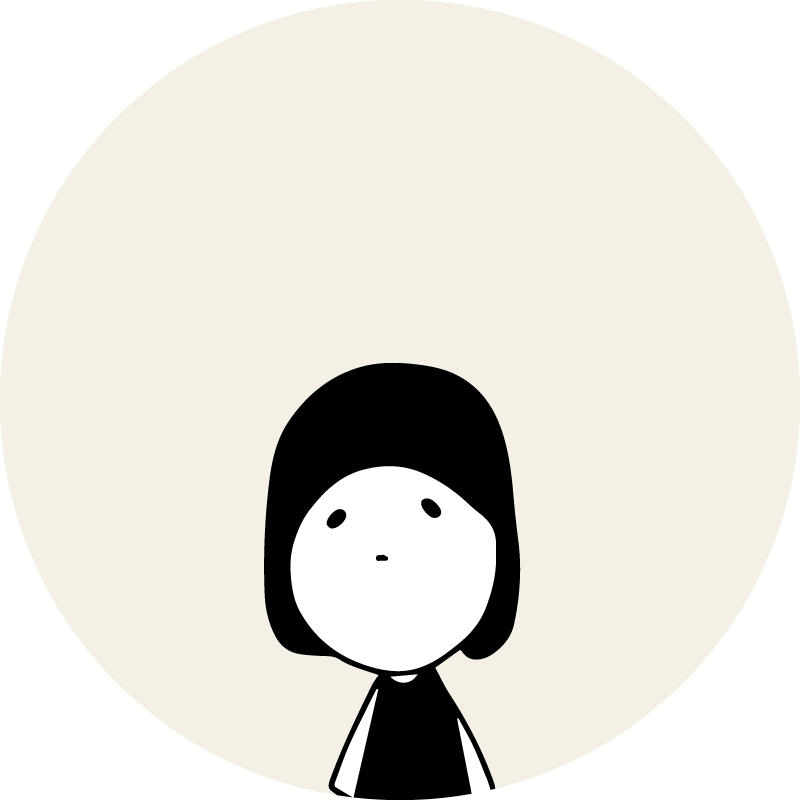